使用基于Net Core的C#编写串口通信程序。串口通信依赖于WiringPi库,所以需要将WiringPi库libwiringPi.so.2.0拷贝到程序运行目录下面。
先去https://github.com/tronsoft/WiringPiSharp下载 WiringPiSharp 。
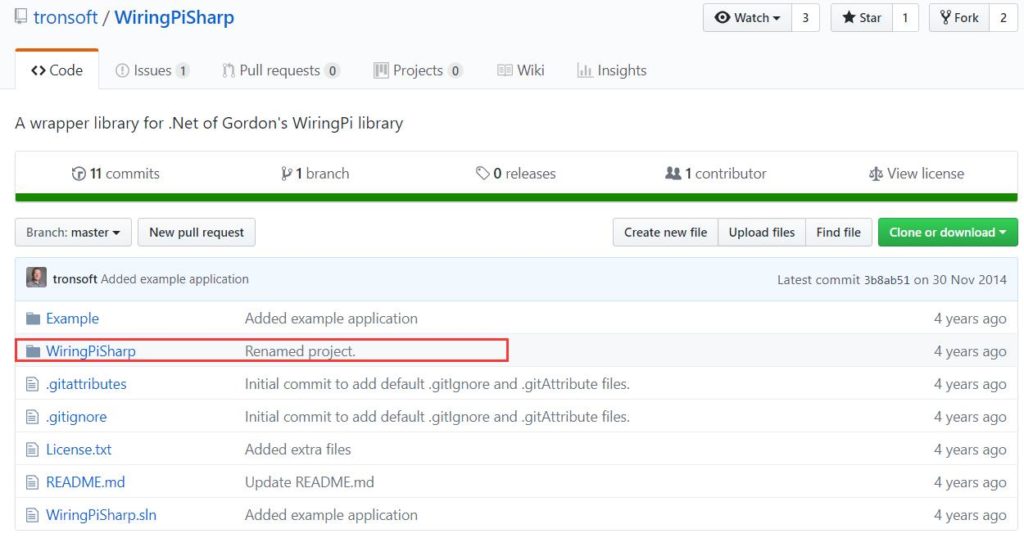
然后将其添加到项目中,并添加引用。
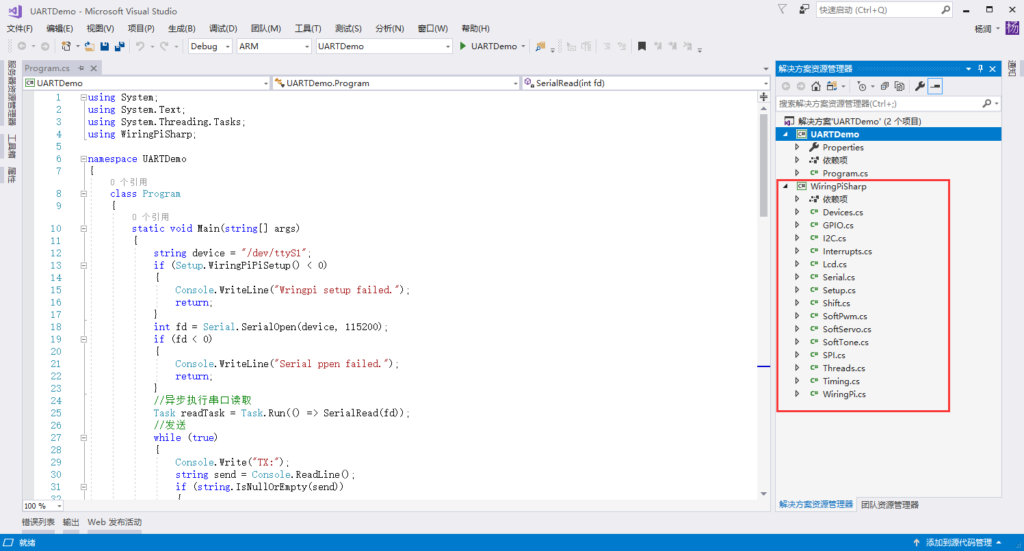
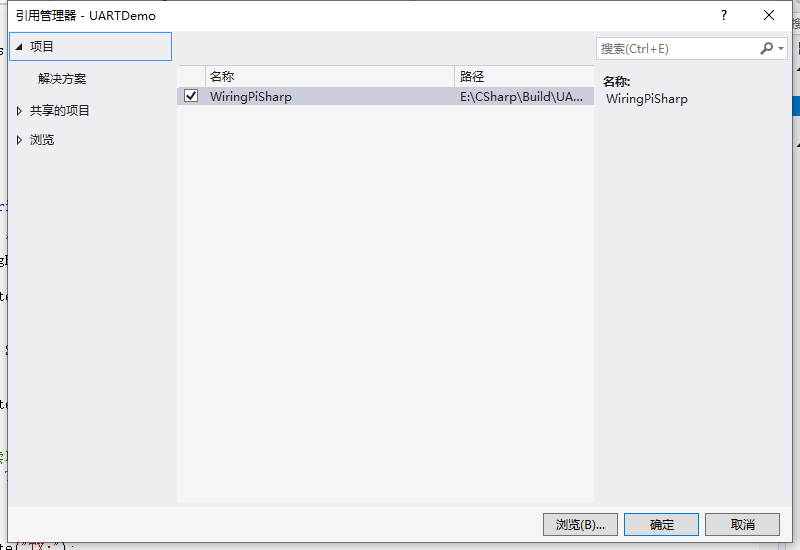
然后编写测试代码
using System;
using System.Text;
using System.Threading.Tasks;
using WiringPiSharp;
namespace UARTDemo
{
class Program
{
static void Main(string[] args)
{
string device = "/dev/ttyS1";
if (Setup.WiringPiPiSetup() < 0)
{
Console.WriteLine("Wringpi setup failed.");
return;
}
int fd = Serial.SerialOpen(device, 115200);
if (fd < 0)
{
Console.WriteLine("Serial ppen failed.");
return;
}
//异步执行串口读取
Task readTask = Task.Run(() => SerialRead(fd));
//发送
while (true)
{
Console.Write("TX:");
string send = Console.ReadLine();
if (string.IsNullOrEmpty(send))
{
continue;
}
if (send == "exit")
{
break;
}
Serial.SerialPuts(fd, send);
}
Serial.SerialClose(fd);
Console.WriteLine("Press any key to exit...");
Console.ReadKey(false);
}
static void SerialRead(int fd)
{
while (true)
{
StringBuilder sb = null;
while (Serial.SerialDataAvail(fd) > 0)
{
int read = Serial.SerialGetchar(fd);
if (read < 0)
{
Serial.SerialFlush(fd);
break;
}
else
{
//Remove r or n
if (read == 10 || read == 13)
{
continue;
}
else
{
if(sb == null)
{
sb = new StringBuilder();
}
sb.Append((char)read);
}
}
}
if (sb != null)
{
string readStr = sb.ToString();
if (!string.IsNullOrWhiteSpace(readStr))
{
Console.WriteLine($"RX:{sb.ToString()}");
}
}
}
}
}
}
程序采用异步执行的方式,在读取数据的同时,可以发送数据。
选择ARM平台发布项目
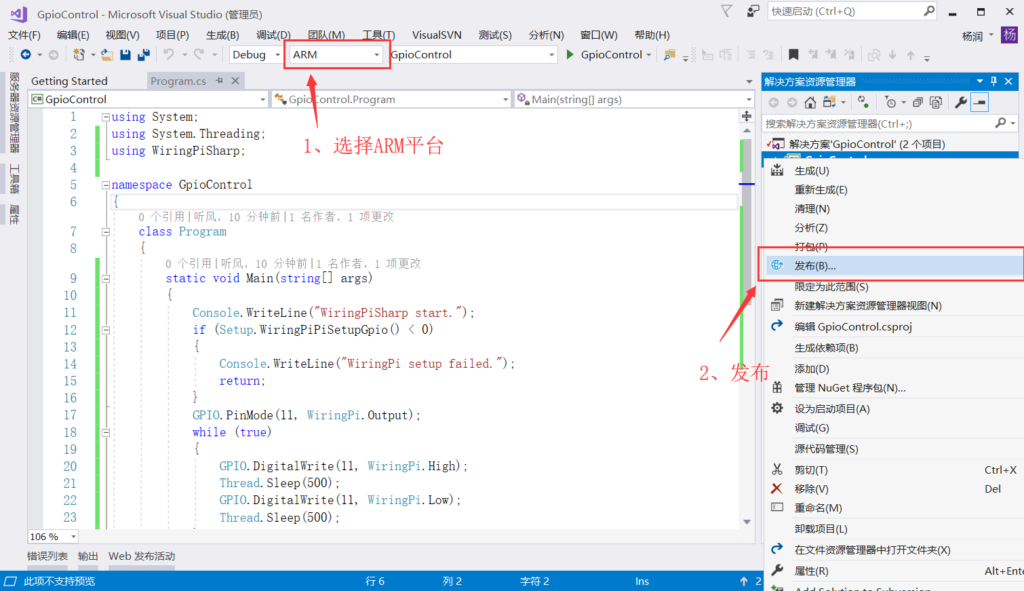
将publish文件夹上传到开发板中,然后将WiringPi的库文件复制到publish当中,程序运行依赖于WiringPi库。手里面的树莓派被我搞坏了,现在用的是Nanopi Duo。
要注意的是,这里我是自己编译的WiringPi,且库文件名必须为libwiringPi.so.2.0。
cp /root/wiringpi/libwiringPi.so.2.0 /publish
然后运行
sudo dotnet UARTDemo.dll
Demo下载
文章评论
您好,ARM平台发布项目怎么创建的?
@我爱阳妈 你好,现在.NET CORE已经原生支持ARM平台的串口通讯了,不建议采用这篇文章里面的方式进行串口通讯,虽然也可以用。原生串口通讯可以参考:https://www.quarkbook.com/?p=712
发布的话,在发布选项卡中选中ARM平台发布就行了。
@管理员 这个是发送字符串的,怎么发送字节数组?叩谢
@我爱阳妈 不建议采用这篇文章里面的方法了,.Net Core在树莓派中可以使用System.IO.Ports下面SerialPort串口类进行串口通信。
SerialPort中的Write方法可以发送字节数组。具体可以查看:https://docs.microsoft.com/zh-cn/dotnet/api/system.io.ports.serialport?redirectedfrom=MSDN&view=dotnet-plat-ext-5.0&viewFallbackFrom=net-5.0
文章的话,可以参考:https://www.quarkbook.com/?p=712